I have been playing with HTML5, CSS and Javascript recently. And I found HTML's Canvas element an amazing option. Its very easy to create animations in canvas. Here's a example of creating Matrix like effect with HTML & Javascript only.
It's looks like attached image. For live demo of the animation visit the link posted at the end.
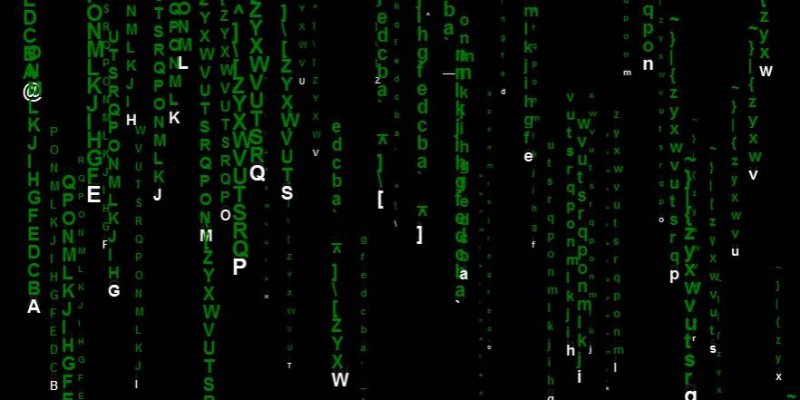
Codes
Explanation:
Its a very basic animation.
1. A 2D array of random character objects is being created. Each object have X & Y coordinates, Speed of animation, color (1st white, rest green), random ASCII character.
2. For each 1D array in the 2D array - all char objects have the same x-coordinate, same speed and li'l decreasing Y-coordinate so that it appears as a vertical line.
3. Each 1D array have increasing X-coordinate to fill up the space horizontally.
4. main animation is done by the Draw function which being called after every 20 milliseconds.
5. The Draw function, each time, paints the canvas black - then draws the characters from the 2D array in defined X-Y coordinates.
6. After each Draw call, the y-coordinate is incremented by the value of "speed" - which ensures next draw will be slightly below the previous position - making it visible like going downward.
7. Once the Y-coordinate reaches the boundary, its made 0 - so that the vertical line reappears from the top.
8. Thats it.
I am new to the HTML5 stuffs. Please pardon the not-so-refined codes.
To check yourself, just copy paste the codes above codes in a HTML page and open in FF or Chrome.
Live demo can be seen my blog post regarding the same.-> HTML5 Canvas : Matrix Effect | pnkj
I don't have much idea about Unicode characters, but if I change the value "ascii_start", in the line
I can see different characters in the Matrix.
Like replacing value of "ascii_start" with 1700 shows some arabic-like characters, 2350 - shows Hindi, 2450 - show Bengali/Assamese characters. (I have no idea whats going on there.
)
It's looks like attached image. For live demo of the animation visit the link posted at the end.
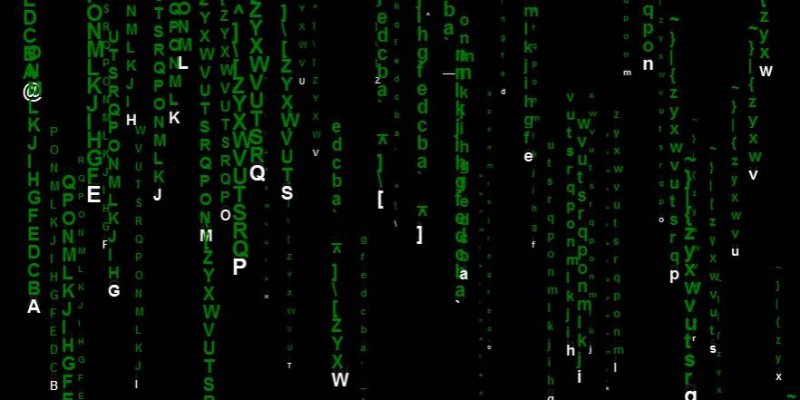
Codes
Code:
<canvas id="canvasId" width="900" height="550" style="border:1px solid black"></canvas>
<script type="text/javascript">
var cW = 900;
var cH = 550;
window.onload = windowReady(64);
/**
windowReady
*/
function windowReady(ascii_start) {
// Load the context of the canvas
var context = document.getElementById('canvasId').getContext("2d");
var charArray = [];
for (var i = 0; i < 75; i++) {
var temp = [];
var yspeed = Math.random() * 10;
var xspacing = Math.random() * 15 + 15;
var fontSize = Math.random() * 20 + 5;
var charlengh = 10+Math.floor(Math.random()*20);
for (var j = 0; j < charlengh; j++) {
temp.push(new create_chars(i * 15 + xspacing, j * 20+20, yspeed, j, fontSize));
}
charArray.push(temp);
}
function create_chars(xloc, yloc, yspeed, j, fontSize) {
this.x = xloc;
this.y = -yloc;
this.dy = yspeed;
this.fontSize = fontSize;
if (j == 0)
this.color = "white";
else
this.color = "green";
this.text = String.fromCharCode(ascii_start + Math.floor(Math.random()*65));
}
function Draw() {
context.globalCompositeOperation = "source-over";
context.fillStyle = "rgba(0,0,0,0.9)";
context.fillRect(0, 0, cW, cH);
context.globalCompositeOperation = "lighter";
for (var k = 0; k < charArray.length; k++) {
for (var m = 0; m < charArray[k].length; m++) {
var charObject = charArray[k][m];
context.fillStyle = charObject.color;
context.font = "bold "+charObject.fontSize+"px Arial";
context.fillText(charObject.text, charObject.x, charObject.y);
context.fill();
charObject.y += charObject.dy;
if (charObject.y > cH) { charObject.y = 0; }
}
}
}
setInterval(Draw, 20);
}
</script>
Explanation:
Its a very basic animation.
1. A 2D array of random character objects is being created. Each object have X & Y coordinates, Speed of animation, color (1st white, rest green), random ASCII character.
2. For each 1D array in the 2D array - all char objects have the same x-coordinate, same speed and li'l decreasing Y-coordinate so that it appears as a vertical line.
3. Each 1D array have increasing X-coordinate to fill up the space horizontally.
4. main animation is done by the Draw function which being called after every 20 milliseconds.
5. The Draw function, each time, paints the canvas black - then draws the characters from the 2D array in defined X-Y coordinates.
6. After each Draw call, the y-coordinate is incremented by the value of "speed" - which ensures next draw will be slightly below the previous position - making it visible like going downward.
7. Once the Y-coordinate reaches the boundary, its made 0 - so that the vertical line reappears from the top.
8. Thats it.
I am new to the HTML5 stuffs. Please pardon the not-so-refined codes.
To check yourself, just copy paste the codes above codes in a HTML page and open in FF or Chrome.
Live demo can be seen my blog post regarding the same.-> HTML5 Canvas : Matrix Effect | pnkj
I don't have much idea about Unicode characters, but if I change the value "ascii_start", in the line
Code:
this.text = String.fromCharCode(ascii_start + Math.floor(Math.random()*65));
Like replacing value of "ascii_start" with 1700 shows some arabic-like characters, 2350 - shows Hindi, 2450 - show Bengali/Assamese characters. (I have no idea whats going on there.
